How to Convert All File Names in a Directory to Lowercase in Linux and MacOS?
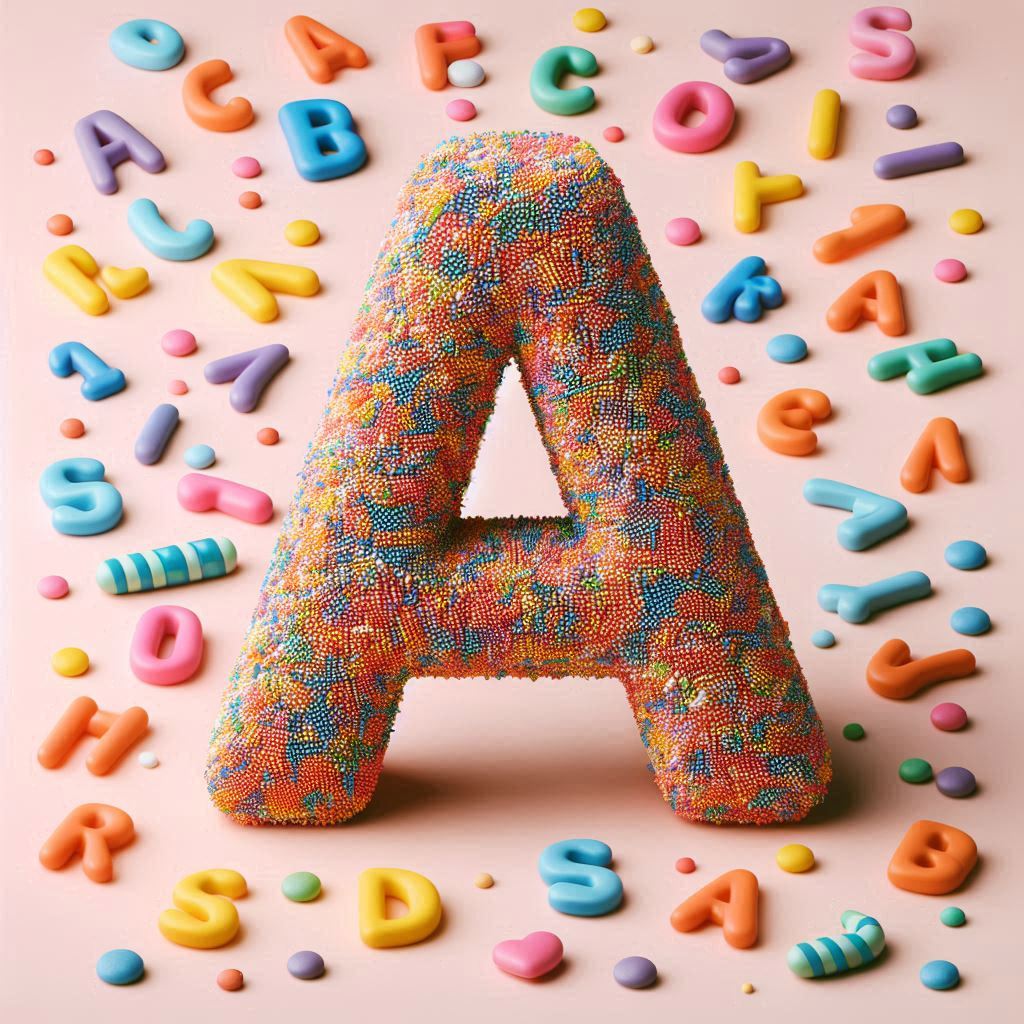
To convert all file names in a folder to lowercase, you can use the following shell script. This script will iterate through each file in the specified directory and rename it to its lowercase equivalent.
Here is a simple script to achieve this:
#!/bin/bash
# Check if a directory argument is provided
if [ -z "$1" ]; then
echo "Usage: $0 /path/to/your/folder"
exit 1
fi
# Assign the first argument to a variable
DIR=$1
# Check if the provided argument is a valid directory
if [ ! -d "$DIR" ]; then
echo "Error: $DIR is not a valid directory."
exit 1
fi
# Navigate to the specified directory
cd "$DIR" || exit
# Loop through all files in the directory
for file in *; do
# Check if the file name is not already in lowercase
lc_file=$(echo "$file" | tr '[:upper:]' '[:lower:]')
if [ "$file" != "$lc_file" ]; then
# Rename the file to its lowercase name
mv "$file" "$lc_file"
fi
done
echo "All file names in $DIR have been converted to lowercase."
Steps to Use the Script
- Save the script to a file, e.g.,
lowercase.sh
. - Give the script execution permissions using the command:
chmod +x lowercase.sh
. - Run the script with the directory path as an argument:
./lowercase.sh /path/to/your/folder
.
Example Usage
If you have a directory /home/user/myfiles
and you want to convert all file names in this directory to lowercase, you would run:
./lowercase.sh /home/user/myfiles
Additional Notes
- This script uses
tr '[:upper:]' '[:lower:]'
to convert file names to lowercase, which is more portable across different Unix-like systems. - Make sure to test the script on a sample directory to ensure it works as expected before using it on important files.